The native Windows Registry API is a C-interface API, that is low-level and kind of hard and cumbersome to use.
For example, suppose that you simply want to read a string value under a given key. You would end up writing code like this:
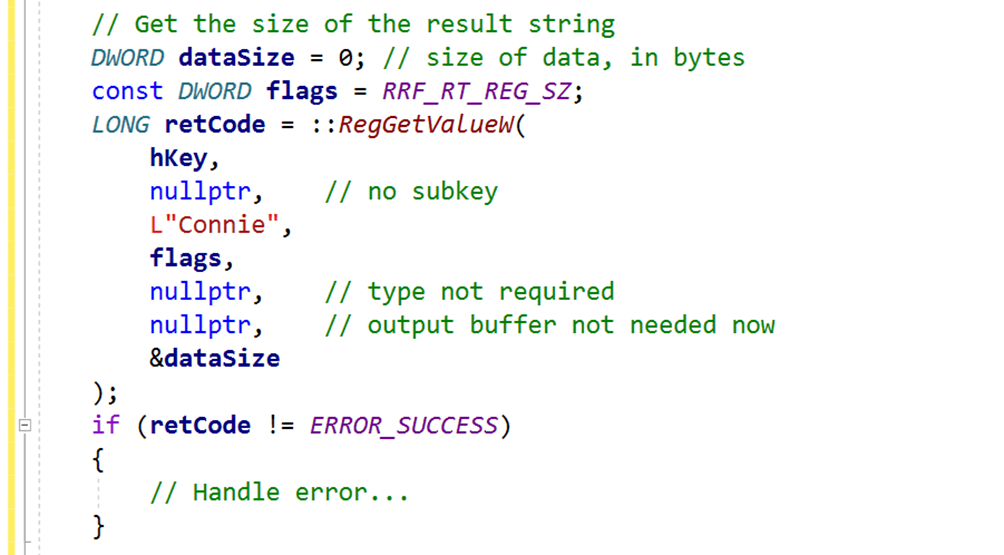
Note how complex and bug-prone that kind of code that directly calls the Windows RegGetValueW API is. And this is just the part to query the destination string length. Then, you need to allocate a string object with proper size (and pay attention to proper size-in-bytes-to-size-in-wchar_ts conversion!), and after that you can finally read the actual string value into the local string object.
That’s definitely a lot of bug-prone C++ code, and this is just to query a string value!
Moreover, in modern C++ code you should prefer using nice higher-level resource manager classes with automatic resource cleanup, instead of raw HKEY handles that are used in the native C-interface Windows Registry API.
Fortunately, it’s possible to hide that kind of complex and bug-prone code in a nice C++ library, that offers a much more programmer-friendly interface. This is basically what my C++ WinReg library does.
For example, with WinReg querying a string value from the Windows Registry is just a simple one-line of C++ code! Look at that:

With WinReg you can also enumerate all the values under a given key with simple intuitive C++ code like this:
auto values = key.EnumValues();
for (const auto & [valueName, valueType] : values)
{
//
// Use valueName and valueType
//
...
}
WinReg is an open-source C++ library, available on GitHub. For the sake of convenience, I packaged and distribute it as a header-only library, which is also available via the vcpkg package manager.
If you need to access the Windows Registry from your C++ code, you may want to give C++ WinReg a try.